Wednesday, 28 June 2017
Angular Js : The Web App Specialist
AngularJS Features
AngularJS is most powerful light weighted JavaScript framework by the web developers and designers developed by google developes team. we hope your going to do well after reading this post, mostly you will learn the main important features of AngularJS. we have covered all the major features of angularjs.
AngularJS Features Index List
- Angularjs Modules
- Angularjs Directives
- Angularjs Templates
- Angularjs Scope
- Angularjs Expressions
- Angularjs Data Binding
- Angularjs MVC (Model-View-Controller)
- Angularjs Validations
- Angularjs Filters
- Angularjs Services
- Angularjs Routing
- Angularjs Dependency Injection
- Angularjs Testing
Modules
AngularJS modules divides your application into modules, so that you can manage things easily, reusable and functional components which can be integrated with other web applications. Each module has a unique name and it can be dependent on other modules.
Creating an AngularJS module
<script type="text/javascript"> angular.module('testApp',[]); angular.module('testApp',['dependentModule1','dependentModule2']); </script>
Using an AngularJS module into your app
You can call your app by using your angularJS module as given below, we have ng-app name called testApp. with this name you can bootstrap your app
<html ng-app="testApp">
<head>...</head>
<body>...</body>
</html>
Inside the model commponent we can define below following components
- Angularjs Controllers
- Angularjs Services
- Angularjs Factories
- Angularjs Filters
- Angularjs Directives etc.
Directives
AngularJS directives works with HTML to extend functionality and to decorate html elements with new behaviors and help to manipulate html elements attributes in interesting way. There are some built-in directives provided by angularjs like asng-app, ng-controller, ng-repeat, ng-model, ng-service etc. You can also create your own custom directive.
Using ng-repeat in Template
ng-repeat will repeats the element based on your requirement. here we have sample app with some products which we need to repeat. look at the folowing ng-repeat code.
<!-- ng-repeat directive --> <ul ng-controller='sampleController'> <li ng-repeat=car in cars'>{{car.name}} = {{car.price}} </li> </ul> <script> function sampleController($scope){ $scope.cars = [ {name: 'Maruti', price: $920.00}, {name: 'SKODA', price: $10000.00}, {name: 'BMW', price: $6000.00}]; } </script>
Angularjs Templates
AngularJS templates are to create induvidual pages, that can render into single page. AngularJS used these templates to show information from the model and controller. to read more about SPA-Single Page Application view this link.
Inside your angularjs templates you can define following angular elements and attributes
- Directive
- Angular Markup ({{}})
- Filters
- Form controls
Creating Template
<html ng-app><!-- Body tag with ngController directive --> <body ng-controller="MyController"> <input ng-model="foo" value="bar"> <!-- Button tag with ng-click directive, and string expression 'buttonText' is wrapped in "{{ }}" markup --> <button ng-click="changeFoo()"> {{buttonText}} </button> <script src="angular.js"> </body>
</html>
Angularjs Expression
Angular expressions are out put for your html code {{ expression }}. Expression are view for html and written inside the currly bracess. These supports filters to format data before displaying it. There are some valid angularjs expressions:
{{ 10 + 5 }} {{ a + b }} {{ a == b }} {{ x = 4 }} {{ user.Id }} {{ items[index] }}
Angularjs Data Binding
Data-Binding: – TWD (two-way data binding) main approach is Automatic synchronization of data between model and view components. to read more about two-data-binding click here. AngularJS data-binding is the most useful feature which save you from writing a considerable amount of boilerplate code. Now, developers are not responsible for manually manipulating the DOM elements and attributes to reflect model changes. AngularJS provides two-way data-binding to handle the synchronization of data between model and view. The data-binding directives helps you to bind your model data to your app view. Use ng-model directive to create a two way data-binding between the input element and the target model.
<div ng-controller='testController'> <input ng-model='name'/><!-- two way --> <p>Hello {{name}}</p> <!-- one way --> </div> <script>function testController($scope){ $scope.name = 'online tutorials from ustutorials.com' } </script>
Angularjs Scope
Scope is an object that refers to the application model. It acts as a context for evaluating expressions. Typically, it acts as a glue between controller and view. Scopes are hierarchical in nature and follow the DOM structure of your angularjs app. Each angular app has a single scope ($rootscope) which mapped to the ng-app directive element.
Creating $scope in controllers
<script> function ProductController($scope){ $scope.Products = [ {name: 'Soap', price: 120}, {name: 'Shampoo', price: 100}, {name: 'Hair Oil', price: 60} ];} </script>
Using Scope in Template
<!-- ng-repeat directive --> <ul ng-controller='ProductController'> <li ng-repeat='product in Products'>{{product.name}} = {{product.price}} </li> </ul>
MVC (Model, View & Controller)
AngularJS is a MVC framework. It does not implement MVC in the traditional way, but rather something closer to MVVM Model-View-ViewModel).
The Model
Models are plain old JavaScript objects that represent data used by your app. Models are also used to represent your app's current state.
The ViewModel
A viewmodel is an object that provides specific data and methods to maintain specific views. Basically, it is a $scope object which lives within your angularjs app's controller. A viewmodel is associated with a HTML element with the ng-model & ng-bind directives.
Creating an AngularJS Controller with ViewModel
<script type="text/javascript"> //defining main controller app.controller('testController', ['$scope', function($scope) { //defining car viewmodel $scope.car = { id: 1, name: 'AngularJS Features', author: 'Jhon KP', }; }]); </script>
The angularjs Controller
The angularjs controller define the actual behavior of your app. It contains business logic for the view and connects the right models to the right views. A controller is associated with a HTML element with the ng-controller directive.
The angularjs View
The view is responsible for presenting your models data to end user. Typically it is the HTML markup which exists after AngularJS has parsed and compiled the HTML to include rendered markup and bindings.
Using an AngularJS controller into your app
<!-- The View --> <div ng-controller="testController"> Id: <span ng-bind="car.id"></span> Name:<input type="text" ng-model="car.name" /> Model: <input type="text" ng-model="car.model" /> </div>
Angularjs Validation
AngularJS also do the form validation, its enables you to develop a modern HTML5 form that is interactive and responsive. AngularJS provides you built-in validation directives to achieve form validation and they are based on the HTML5 form validators. You can also create your own custom validators.
Here is a list of angularjs directive which can be apply on a input field to validate it's value.
<inputtype="text" ng-model="{ string }" [name="{ string }"[ [ng-required="{ boolean }"] [ng-minlength="{ number }"] [ng-maxlength="{ number }"] [ng-pattern="{ string }"] [ng-change="{ string }"]> </input>
Angularjs Filter
Angularjs Filters are used to format and filter data before displaying it to the user. They can be used in view templates, controllers, services and directives. There are some built-in filters provided by angularjs like as Currency, Date, Number, OrderBy, Lowercase, Uppercase etc. You can also create your own filters.
Filter Syntax
{{ expression | filter}}
Filter Example
<script type="text/javascript">
{{ 14 | currency }} //returns $14.00
</script>
Angularjs Services
Services are reusable singleton objects that are used to organize and share code across your app. They can be injected into controllers, filters, directives.
Angularjs $http Service
<script> $http.get('/products') .success(function(data) { $scope.products = data; }); }); </script>
AngularJS offers several built-in services (like $http, $provide, $resource,$window,$parse) which always start with $ sign. You can also create your own services
Angularjs Routing
AngularJS Routing helps you to divide your app into multiple section views and bind different views to Controllers. The magic of Routing is taken care by a angularjs service $routeProvider. $routeProvider service provides method .when() and .otherwise() to define the routes for your app. Routing has dependency on ngRoute module.
<script type="text/javascript"> var testApp = angular.module('testApp', ['ngRoute']); testApp.config(['$routeProvider', function($routeProvider) { $routeProvider. when('/products', { //route templateUrl: 'templates/products.html', controller: 'testController' }). when('/product/:productId', { //route with parameter templateUrl: 'templates/product.html', controller:'testController'}). otherwise({ //default route redirectTo: '/index' }); }]); </script>
Angularjs Dependency Injection
There are following three ways of injecting dependencies into your code:?
Implicitly from the function parameter names
<script type="text/javascript"> function testController($scope, greeter) { } </script>
Using the $inject property annotation
<script type="text/javascript"> var testController = function(renamed$scope, renamedGreeter) { } testController['$inject'] = ['$scope', 'greeter']; </script>
Using the inline array annotation
<script type="text/javascript"> someModule.factory('greeter', ['$window', function(renamed$window) { }]);
</script>
Tuesday, 6 June 2017
DevOps : Evolution ( Introduction Part 3)
![The Evolution of DevOps: Enterprise’s Solution to Software Development [Infographic]:](https://s-media-cache-ak0.pinimg.com/564x/4f/73/a3/4f73a317e9ea09fcc40f992414ba76da.jpg)
DevOps is the combination of cultural philosophies, practices, and tools that increases an organization’s ability to deliver applications and services at high velocity: evolving and improving products at a faster pace than organizations using traditional software development and infrastructure management processes. This speed enables organizations to better serve their customers and compete more effectively in the market.
- This model is simple and easy to understand and use.
- It is easy to manage due to the rigidity of the model – each phase has specific deliverables and a review process.
- In this model phases are processed and completed one at a time. Phases do not overlap.
- Waterfall model works well for smaller projects where requirements are very well understood.
- Once an application is in the testing stage, it is very difficult to go back and change something that was not well-thought out in the concept stage.
- No working software is produced until late during the life cycle.
- High amounts of risk and uncertainty.
- Not a good model for complex and object-oriented projects.
- Poor model for long and ongoing projects.
- Not suitable for the projects where requirements are at a moderate to high risk of changing.
- This model is used only when the requirements are very well known, clear and fixed.
- Product definition is stable.
- Technology is understood.
- There are no ambiguous requirements
- Ample resources with required expertise are available freely
- The project is short.
DevOps :Introduction
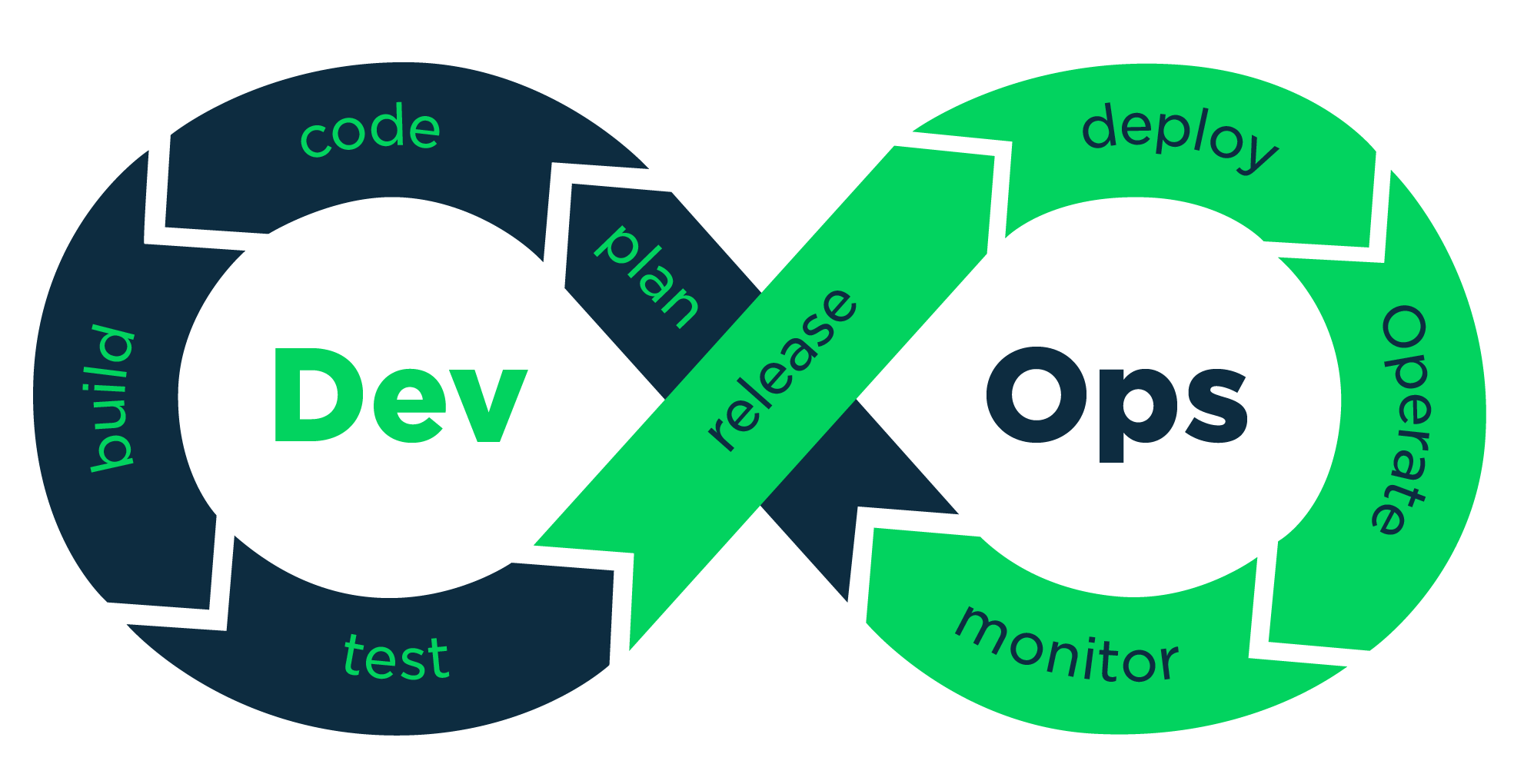
1.Continuous integration
Abstract Class & Interface in Java
Abstract Class & Interface in Java Here is the most important topic on Java Abstract class and interface, Abstraction ...

-
Constructors in Java Constructor in java is a special type of method that is used to initialize the object. Java constructor is invok...
-
Abstract Class & Interface in Java Here is the most important topic on Java Abstract class and interface, Abstraction ...
-
Inheritance One of the most Basic building blocks of object oriented Programming is inheritance with t...